All Things Arel Basics - Flagrant
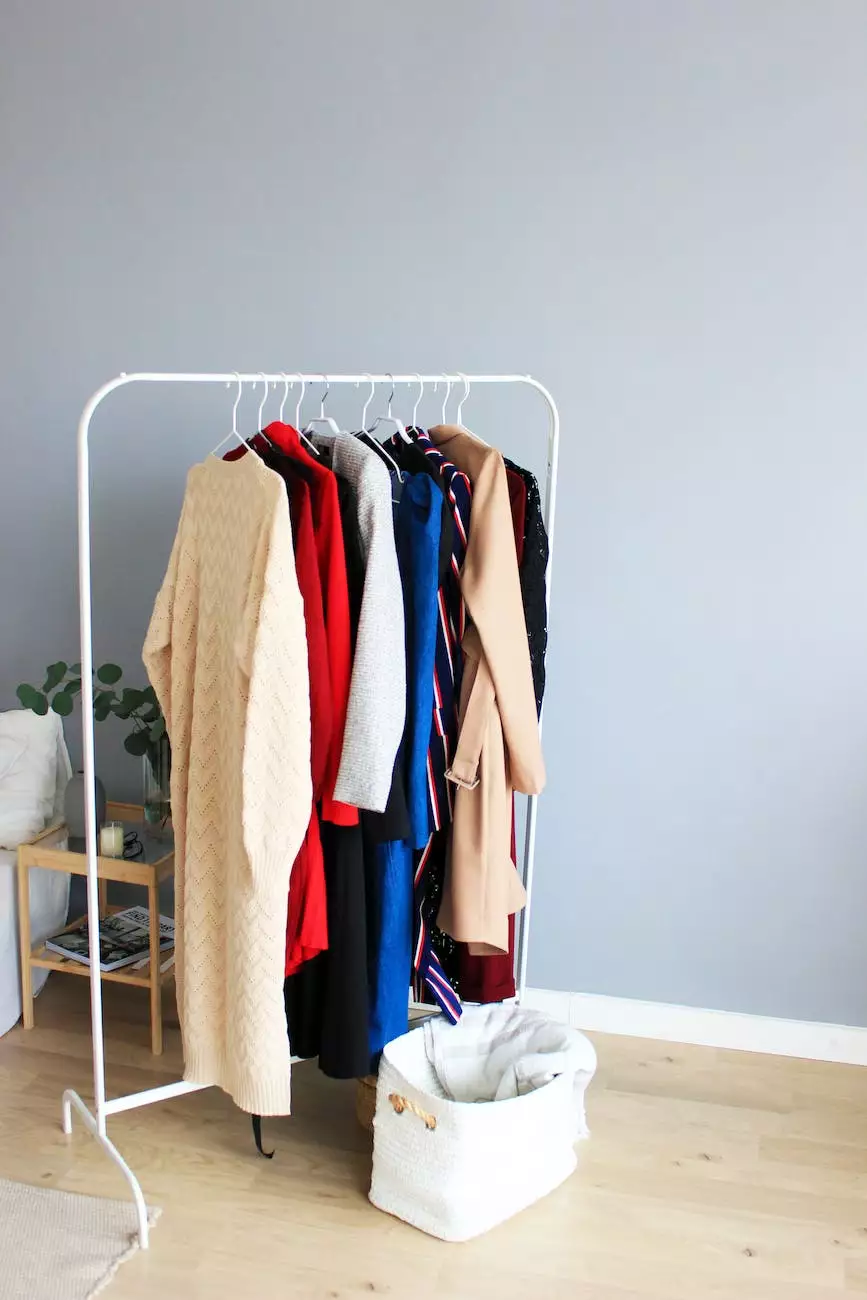
Introduction
Welcome to RPM Design and Prototype's comprehensive guide on Arel basics. In this article, we will dive into the world of Arel, an object relational mapper (ORM) for Ruby, and help you understand its powerful features and capabilities.
What is Arel?
Arel is a querying interface for relational databases in Ruby. It provides a clean and intuitive way to construct complex database queries using object-oriented syntax. With Arel, you can dynamically generate SQL queries without relying solely on raw SQL strings, making your code more readable, maintainable, and less prone to SQL injection attacks.
The Power of Arel
Arel offers a wide range of features that empower developers to build advanced and efficient database queries. Let's explore some of its key capabilities:
1. Query Composition
Arel allows you to compose complex queries by chaining methods together. It provides a fluent interface that enables you to easily add conditions, joins, and orderings to your queries. This flexibility makes it simple to construct queries for even the most intricate database relationships.
2. Database Abstraction
With Arel, you can write database-agnostic queries. It abstracts away the differences between different database management systems (DBMS) and provides a unified interface for querying across multiple database backends, such as MySQL, PostgreSQL, and SQLite. This abstraction makes it easier to switch between different DBMSs without rewriting your queries.
3. Advanced Join Capabilities
Arel offers powerful join capabilities, including inner joins, outer joins, and even more complex join types like left outer joins and right outer joins. These join capabilities enable you to combine data from multiple tables and retrieve exactly the information you need for your applications.
4. Dynamic Query Generation
One of the greatest strengths of Arel is its ability to dynamically generate queries based on runtime conditions. This enables you to construct queries that adapt to changing requirements, such as user input or business logic. Arel's dynamic query generation helps you write cleaner, more flexible, and future-proof code.
5. SQL Function Support
Arel comes with extensive support for various SQL functions, allowing you to perform complex calculations and aggregations within your queries. Functions like sum, count, average, and many others are readily available, allowing you to harness the full power of your database engine.
Arel in Action
Let's explore a practical use case to see how Arel can simplify your database queries. Consider a scenario where you need to retrieve all orders with their corresponding products, but only for active customers and within a specific date range.
Step 1: Setting up the Environment
Before we dive into the example, make sure you have Arel installed in your Ruby environment. Include the necessary dependencies and set up your database connection accordingly.
Step 2: Constructing the Query
Using Arel, we can build the query in a modular and readable way:
# Initialize the base query orders = Order.arel_table # Define the necessary joins join_customer = orders.join(Customers.arel_table).on(orders[:customer_id].eq(customers[:id])) join_product = orders.join(Products.arel_table).on(orders[:product_id].eq(products[:id])) # Apply conditions query = orders .join(join_customer) .join(join_product) .where(orders[:order_date].between('2021-01-01').and('2021-12-31')) .where(customers[:status].eq('active')) # Fetch the results results = Order.find_by_sql(query.to_sql)Step 3: Analyzing the Code
In this example, we initialize the base query using the Arel table for the 'orders' table. We then define the necessary joins with the 'customers' and 'products' tables based on their respective foreign key relationships.
Next, we apply the desired conditions to our query. We restrict the order date to be within a specific date range using the 'between' operator and additionally filter for active customers using the 'eq' (equals) operator.
Finally, we execute the query and retrieve the results using ActiveRecord's 'find_by_sql' method. The resulting records contain the desired information, including the joined customer and product data.
Step 4: Testing and Optimizing
Once you have constructed your query, it's crucial to test and optimize it for performance. Measure its execution time, analyze the generated SQL, and consider adding appropriate indexes to enhance query performance.
Conclusion
Congratulations! You now have a solid understanding of the Arel basics and its powerful features. By utilizing Arel in your Ruby projects, you can write cleaner, more expressive, and more efficient database queries. Dive deeper into Arel's documentation and explore advanced topics to unlock its full potential.
If you have any questions or need further assistance, don't hesitate to reach out to the RPM Design and Prototype team. Happy coding!